In this post, I'll guide you through updating your Aspire application to the latest .NET Aspire Preview 3 and incorporating an NPM project, whether it's Angular, React, Vue, or in my case, Qwik. Ensure you have the most recent .NET SDK and Visual Studio 2022 Preview installed before proceeding.
Install Prerequisites:
- Ensure you have:
- Latest .NET SDK
- Visual Studio 2022 Preview
- From Visual Studio Installer, install .NET Aspire SDK (Preview) by navigating to:
Modify -> Individual Components -> .NET Aspire SDK (Preview)
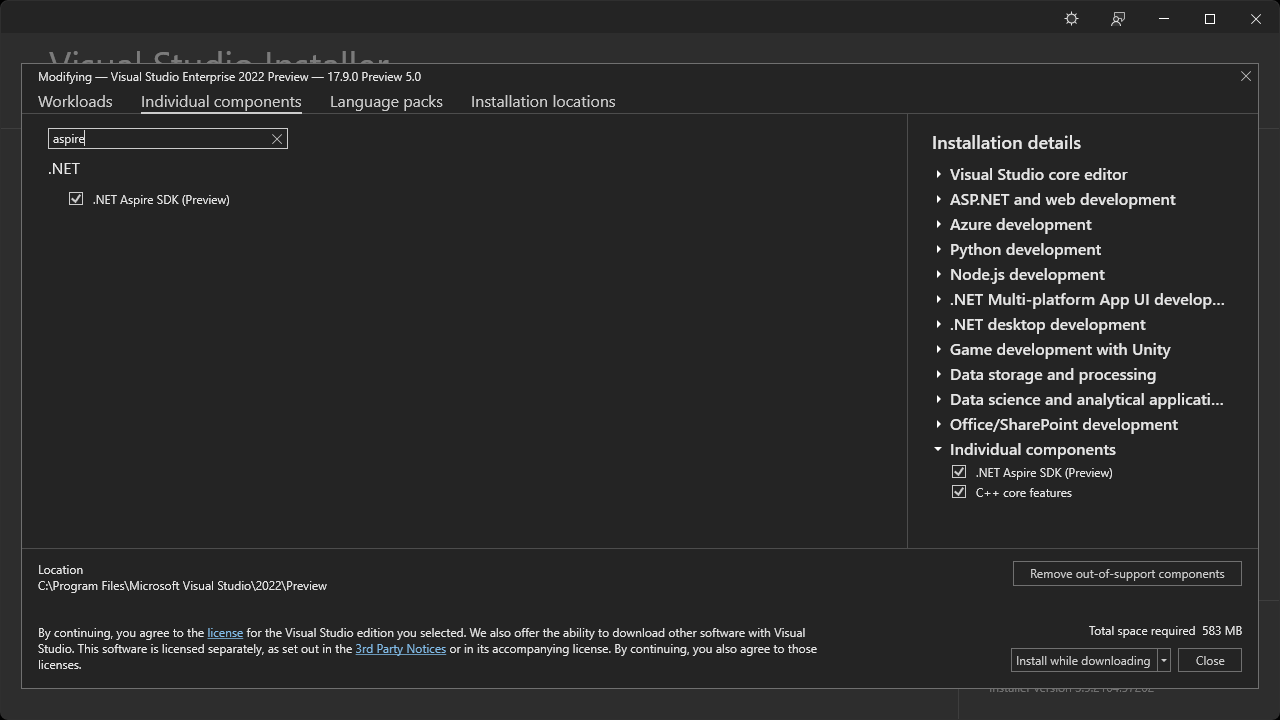
Update .NET Aspire Workload:
- Update the .NET Aspire workload via terminal with the following commands:
From a terminal, run the following commands to update the .NET Aspire workload,
dotnet workload update
dotnet workload install aspire
Create a New .NET Aspire Starter Application:
- Start with a fresh .NET Aspire Starter Application.
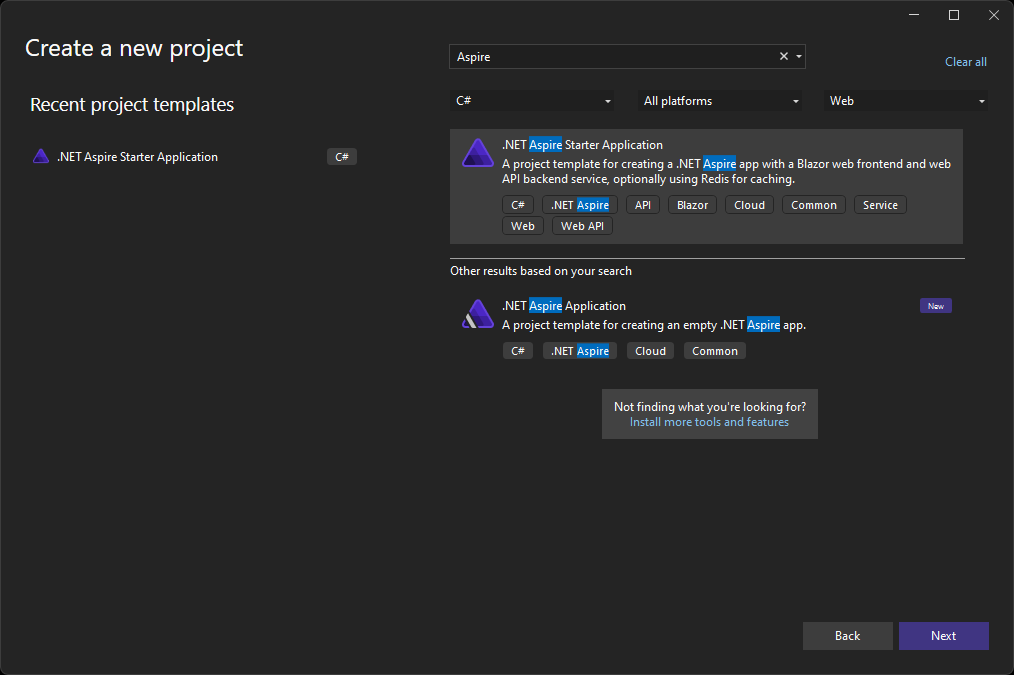
Update Existing Apps:
- For existing .NET Aspire apps, after installing the latest workload, update all .NET Aspire package references to:
8.0.0-preview.3.24105.21
- For example, update package references in the
SampleAspireApp.AppHost.csproj
file for Aspire.Hosting.
<PackageReference Include="Aspire.Hosting" Version="8.0.0-preview.3.24105.21" />
Bootstrap Qwik Application:
- Change directory to the created Aspire project in your terminal.
- Use the following command to create a Qwik project, specifying the directory name for the project files.
npm create qwik@latest
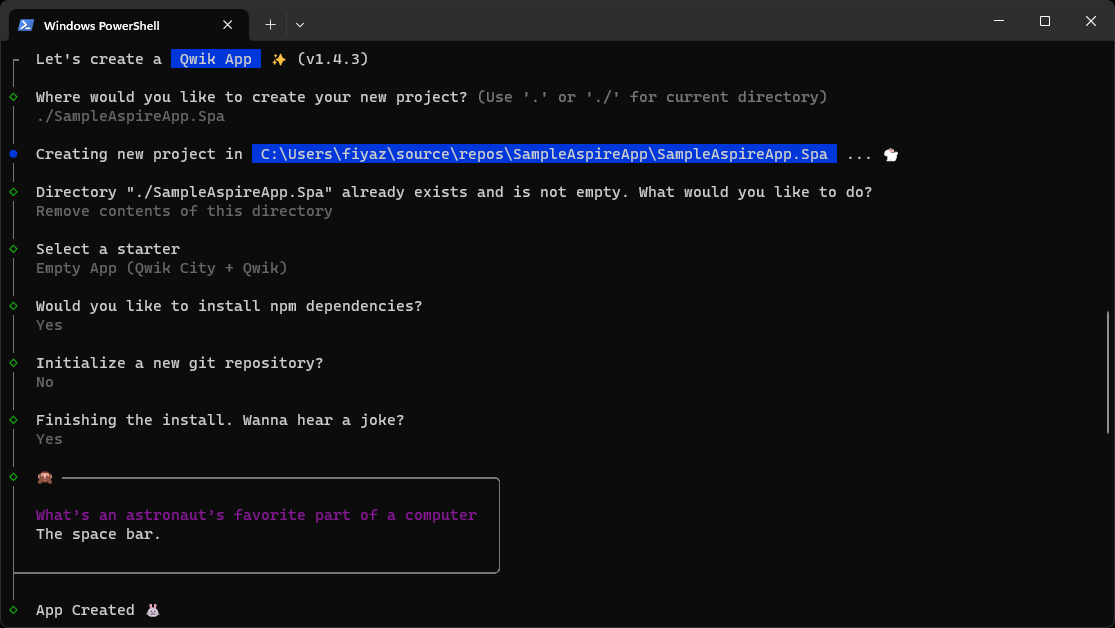
Add Dockerfile and Environment Variables:
- Add a Dockerfile to the Qwik project.
FROM node:20.7
WORKDIR /app
COPY package.json package.json
COPY package-lock.json package-lock.json
RUN npm i
COPY . .
EXPOSE 5173
CMD [ "npm", "start" ]
- Also, include a
.env
file with the environment variable pointing to the Aspire ApiService project running on the Docker container.
PUBLIC_QWIK_APP_WEATHER_API=$services__apiservice__1
Update Program.cs File:
- Add the following to your
Program.cs
file in the AppHost project:
builder.AddNpmApp("qwik", "../SampleAspireApp.Spa")
.WithReference(apiservice)
.WithHttpEndpoint(containerPort: 5173, env: "PORT")
.AsDockerfileInManifest();
- Use
AddNpmApp
to incorporate the Qwik app, specifying the node project name and path. - Set the container port to 5173, meaning the Qwik app will be hosted on
http://localhost:5173
.
Final Touches:
- Run the Aspire project, and two browser windows should pop up.
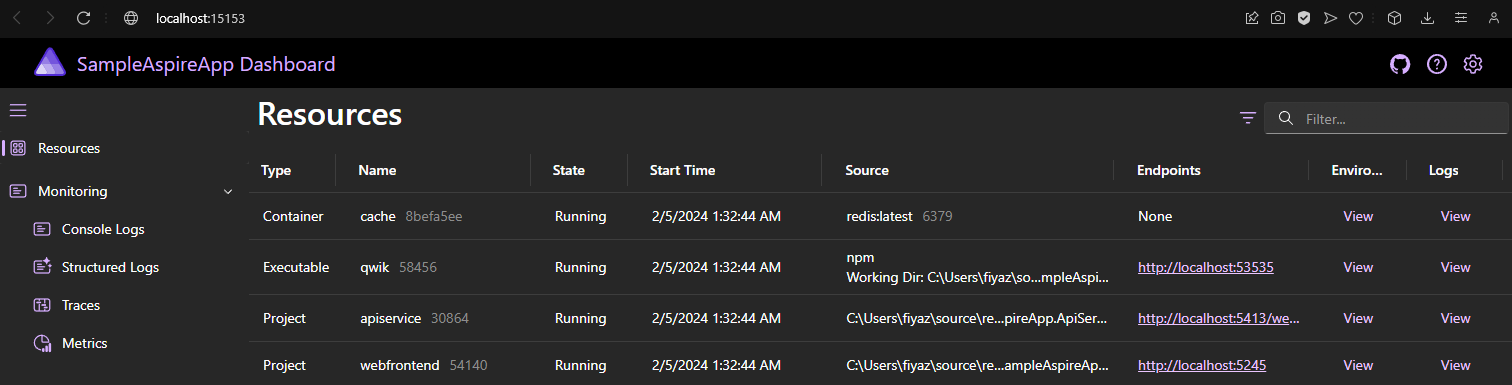

Fetching Forecast Data:
- Fetch the forecast from the ApiService and display it in the UI by adding the necessary code to the
index.tsx
file.
import { Resource, component$, useResource$ } from "@builder.io/qwik";
import type { DocumentHead } from "@builder.io/qwik-city";
export default component$(() => {
const forecast = useResource$<Forecast[]>(async () => {
const response = await fetch(
`${import.meta.env.PUBLIC_QWIK_APP_WEATHER_API}/weatherforecast`
);
const data = await response.json();
return data as Forecast[];
});
return (
<>
<Resource
value={forecast}
onPending={() => <p>Loading...</p>}
onResolved={(forecast: Forecast[]) => <table>
<thead>
<tr>
<th>Date</th>
<th>Temp. (C)</th>
<th>Temp. (F)</th>
<th>Summary</th>
</tr>
</thead>
<tbody>
{forecast.map((f: Forecast, i: number) => {
return (
<tr key={i}>
<td>{f.date}</td>
<td>{f.temperatureC}</td>
<td>{f.temperatureF}</td>
<td>{f.summary}</td>
</tr>
);
})}
</tbody>
</table>}
/>
</>
);
});
type Forecast = {
date: string;
temperatureC: number;
temperatureF: number;
summary: string;
}
export const head: DocumentHead = {
title: "Welcome to Qwik",
meta: [
{
name: "description",
content: "Qwik site description",
},
],
};
Check Results:
- Ensure the app displays the forecast in a styled table.
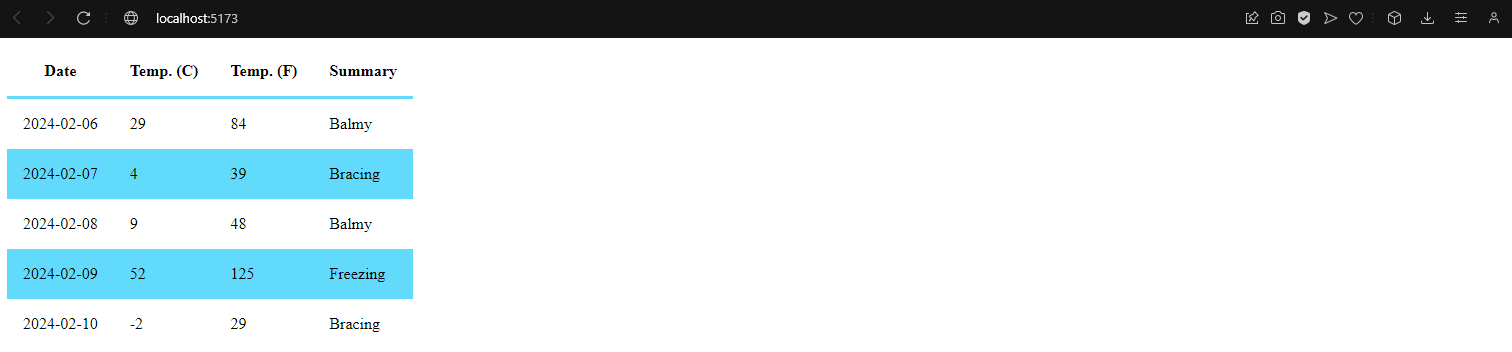
Resources:
- For reference, you can find the complete repository and important links below.
That's it! You've successfully updated your Aspire application and integrated a Qwik project. Enjoy exploring the possibilities of your enhanced application.
Comments